C++ is a powerful language, but it can also be slow. If you’re not careful, your C++ code can slow down your application.
There are a number of performance issues that can affect C++ code. In this blog post, we’ll discuss the 5 biggest performance issues in C++ and how to fix them.
By avoiding these performance issues, you can improve the performance of your C++ applications and provide a better user experience.
Let’s get started!
Inefficient algorithms
Using inefficient algorithms can lead to significant performance problems. For example, using a bubble sort algorithm to sort a large list is much slower than using a quicksort algorithm.
// This code uses a bubble sort algorithm to sort a large list. // Bubble sort is an inefficient algorithm, and this code will be slow. int list[] = {1, 5, 3, 2, 4}; for (int i = 0; i < sizeof(list) / sizeof(list[0]); i++) { for (int j = 0; j < sizeof(list) / sizeof(list[0]) - 1; j++) { if (list[j] > list[j + 1]) { int temp = list[j]; list[j] = list[j + 1]; list[j + 1] = temp; } } }
Memory leaks
Memory leaks can cause your application to run out of memory, leading to performance problems. It is important to free any memory that you no longer need.
// This code leaks memory. // The `string` object created in the `f()` function is never deleted, // so it will eventually leak memory. void f() { string str = "Hello, world!"; } int main() { f(); return 0; }
Unnecessary function calls
Making unnecessary function calls can slow down your application. For example, if you have a function that only performs a simple operation, you can avoid making a function call by performing the operation directly.
// This code makes unnecessary function calls. // The `sqrt()` function is only called once, even though it could be called // multiple times. double area = sqrt(pow(x, 2) + pow(y, 2));
Poorly written code
Poorly written code can be slow. For example, if you have a lot of nested loops, your code will be slower than if you used a more efficient data structure.
// This code is poorly written. // The `for` loop is nested inside the `while` loop, which is inefficient. int i = 0; while (i < 10) { for (int j = 0; j < 10; j++) { // ... } i++; }
Unoptimized code
Even if your code is well-written, it may not be optimized for performance. For example, you can use compiler flags to optimize your code for speed.
// This code is not optimized for performance.
// The `strlen()` function could be replaced with a simple `sizeof()` operation.
int len = strlen(“Hello, world!”);
How to Improve the Performance of Your C++ Applications with FusionReactor
FusionReactor is an observability platform that can collect, store, and visualize telemetry data. FusionReactor offers a number of features that are similar to Grafana, including:
- Data collection: FusionReactor can collect telemetry data from various sources, including Prometheus, OpenTelemetry, and Graphite.
- Data storage: FusionReactor can store telemetry data in various formats, including JSON, CSV, and Prometheus.
- Data visualization: FusionReactor can visualize telemetry data in a variety of charts and graphs.
- Alerting: FusionReactor can send alerts when telemetry data exceeds certain thresholds.
- Dashboards: FusionReactor can create dashboards that display telemetry data in a way that is easy to understand.
Overall, FusionReactor is a powerful monitoring and visualization platform that can be used to collect, store, and visualize telemetry data. FusionReactor offers a number of features that are similar to Grafana, and it can be a good substitute for Grafana in some cases.
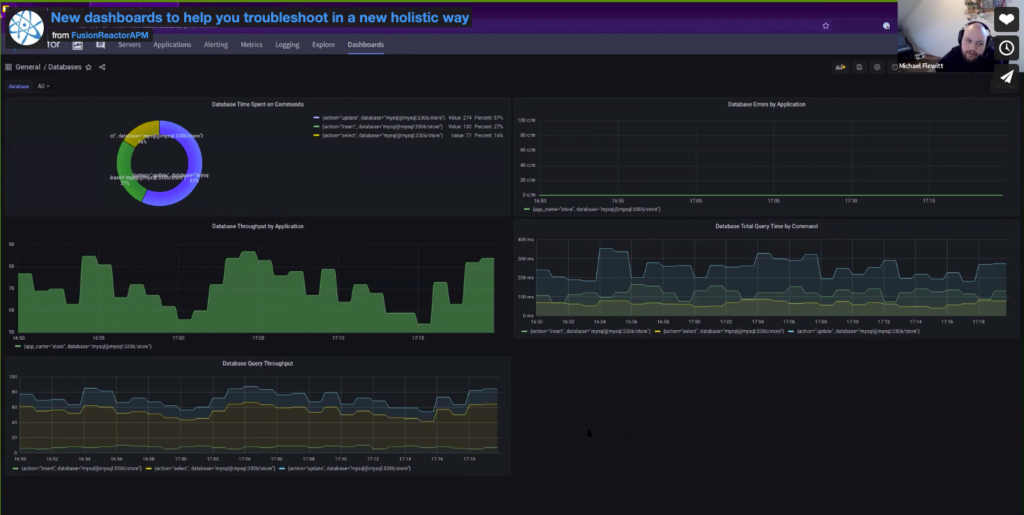
Advantages of FusionReactor
- Flexible: FusionReactor can be used to collect, store, and visualize telemetry data from a variety of sources.
- Scalable: FusionReactor can be scaled to meet the needs of large-scale applications.
- Extensible: FusionReactor can be extended with plugins to add new features.
- Low-cost: Compared to its rivals FusionReactor offers all the essential tools you need at an affordable price
- A number of features, including log monitoring, distributed tracing, infrastructure monitoring, error tracking and a number of profilers
Overall, FusionReactor is a powerful monitoring and visualization platform that can be used to collect, store, and visualize telemetry data. FusionReactor offers a number of features that are similar to Grafana but without the complex configuration.